The purpose of this book is to give the reader a better understanding of how computers really work at a lower level than in programming languages like Pascal. By gaining a deeper understanding of how computers work, the reader can often be much more productive developing software in higher level languages such as C and C++. Learning to program in assembly language is an excellent way to achieve this goal. Other PC assembly language books still teach how to program the 8086 processor that the original PC used in 1981! The 8086 processor only supported real mode. In this mode, any program may address any memory or device in the computer. This mode is not suitable for a secure, multitasking operating system. This book instead discusses how to program the 80386 and later processors in protected mode (the mode that Windows and Linux runs in). This mode supports the features that modern operating systems expect, such as virtual memory and memory protection.
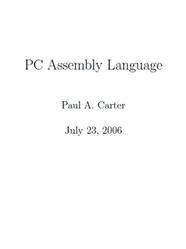
The CPU.
The Central Processing Unit (CPU) is the physical device that performs instructions. The instructions that CPUs perform are generally very simple. Instructions may require the data they act on to be in special storage locations in the CPU itself called registers. The CPU can access data in registers much faster than data in memory. However, the number of registers in a CPU is limited, so the programmer must take care to keep only currently used data in registers.
The instructions a type of CPU executes make up the CPU’s machine language. Machine programs have a much more basic structure than higher-level languages. Machine language instructions are encoded as raw numbers, not in friendly text formats. A CPU must be able to decode an instruction’s purpose very quickly to run efficiently. Machine language is designed with this goal in mind, not to be easily deciphered by humans. Programs written in other languages must be converted to the native machine language of the CPU to run on the computer. A compiler is a program that translates programs written in a programming language into the machine language of a particular computer architecture. In general, every type of CPU has its own unique machine language. This is one reason why programs written for a Mac can not run on an IBM-type PC.
CONTENTS.
Preface.
1 Introduction.
1.1 Number Systems.
1.1.1 Decimal.
1.1.2 Binary.
1.1.3 Hexadecimal.
1.2 Computer Organization.
1.2.1 Memory.
1.2.2 The CPU.
1.2.3 The 80x86 family of CPUs.
1.2.4 8086 16-bit Registers.
1.2.5 80386 32-bit registers.
1.2.6 Real Mode.
1.2.7 16-bit Protected Mode.
1.2.8 32-bit Protected Mode.
1.2.9 Interrupts.
1.3 Assembly Language.
1.3.1 Machine language.
1.3.2 Assembly language.
1.3.3 Instruction operands.
1.3.4 Basic instructions.
1.3.5 Directives.
1.3.6 Input and Output.
1.3.7 Debugging.
1.4 Creating a Program.
1.4.1 First program.
1.4.2 Compiler dependencies.
1.4.3 Assembling the code.
1.4.4 Compiling the C code.
1.4.5 Linking the object files.
1.4.6 Understanding an assembly listing file.
1.5 Skeleton File.
2 Basic Assembly Language.
2.1 Working with Integers.
2.1.1 Integer representation.
2.1.2 Sign extension.
2.1.3 Two’s complement arithmetic.
2.1.4 Example program.
2.1.5 Extended precision arithmetic.
2.2 Control Structures.
2.2.1 Comparisons.
2.2.2 Branch instructions.
2.2.3 The loop instructions.
2.3 Translating Standard Control Structures.
2.3.1 If statements.
2.3.2 While loops.
2.3.3 Do while loops.
2.4 Example: Finding Prime Numbers.
3 Bit Operations 47
3.1 Shift Operations.
3.1.1 Logical shifts.
3.1.2 Use of shifts.
3.1.3 Arithmetic shifts.
3.1.4 Rotate shifts.
3.1.5 Simple application.
3.2 Boolean Bitwise Operations.
3.2.1 The AND operation.
3.2.2 The OR operation.
3.2.3 The XOR operation.
3.2.4 The NOT operation.
3.2.5 The TEST instruction.
3.2.6 Uses of bit operations.
3.3 Avoiding Conditional Branches.
3.4 Manipulating bits in C.
3.4.1 The bitwise operators of C.
3.4.2 Using bitwise operators in C.
3.5 Big and Little Endian Representations.
3.5.1 When to Care About Little and Big Endian.
3.6 Counting Bits.
3.6.1 Method one.
3.6.2 Method two.
3.6.3 Method three.
4 Subprograms.
4.1 Indirect Addressing.
4.2 Simple Subprogram Example.
4.3 The Stack.
4.4 The CALL and RET Instructions.
4.5 Calling Conventions.
4.5.1 Passing parameters on the stack.
4.5.2 Local variables on the stack.
4.6 Multi-Module Programs.
4.7 Interfacing Assembly with C.
4.7.1 Saving registers.
4.7.2 Labels of functions.
4.7.3 Passing parameters.
4.7.4 Calculating addresses of local variables.
4.7.5 Returning values.
4.7.6 Other calling conventions.
4.7.7 Examples.
4.7.8 Calling C functions from assembly.
4.8 Reentrant and Recursive Subprograms.
4.8.1 Recursive subprograms.
4.8.2 Review of C variable storage types.
5 Arrays.
5.1 Introduction.
5.1.1 Defining arrays.
5.1.2 Accessing elements of arrays.
5.1.3 More advanced indirect addressing.
5.1.4 Example.
5.1.5 Multidimensional Arrays.
5.2 Array/String Instructions.
5.2.1 Reading and writing memory.
5.2.2 The REP instruction prefix.
5.2.3 Comparison string instructions.
5.2.4 The REPx instruction prefixes.
5.2.5 Example.
6 Floating Point.
6.1 Floating Point Representation.
6.1.1 Non-integral binary numbers.
6.1.2 IEEE floating point representation.
6.2 Floating Point Arithmetic.
6.2.1 Addition.
6.2.2 Subtraction.
6.2.3 Multiplication and division.
6.2.4 Ramifications for programming.
6.3 The Numeric Coprocessor.
6.3.1 Hardware.
6.3.2 Instructions.
6.3.3 Examples.
6.3.4 Quadratic formula.
6.3.5 Reading array from file.
6.3.6 Finding primes.
7 Structures and C++.
7.1 Structures.
7.1.1 Introduction.
7.1.2 Memory alignment.
7.1.3 Bit Fields.
7.1.4 Using structures in assembly.
7.2 Assembly and C++.
7.2.1 Overloading and Name Mangling.
7.2.2 References.
7.2.3 Inline functions.
7.2.4 Classes.
7.2.5 Inheritance and Polymorphism.
7.2.6 Other C++ features.
A 80x86 Instructions.
A.1 Non-floating Point Instructions.
A.2 Floating Point Instructions.
Index.
Бесплатно скачать электронную книгу в удобном формате, смотреть и читать:
Скачать книгу PC Assembly Language, Carter P.A., 2006 - fileskachat.com, быстрое и бесплатное скачивание.
Скачать pdf
Ниже можно купить эту книгу по лучшей цене со скидкой с доставкой по всей России.Купить эту книгу
Скачать - pdf - Яндекс.Диск.
Дата публикации:
Теги: учебник по программированию :: программирование :: Carter
Смотрите также учебники, книги и учебные материалы:
Следующие учебники и книги:
- Программирование на C++ в Visual Studio 2010 Express, Прохоренок Н.А., 2010
- Практикум по программированию в Турбо Паскале, Анцыпа В.А., Вдовин В.В.
- CSS, Кратко о самом главном, Дуванов А.А., 2009
- UNIX, Программное окружение, Керниган Б., Пайк Р., 2003
Предыдущие статьи:
- Object-Oriented Programming in C++, Lafore R., 2002
- Физика для разработчиков компьютерных игр, Конгер Д., 2007
- Financial Modelling in Python, Fletcher S., Gardner C., 2009
- Программирование на Visual Fortran, Алгазин С.Д., Кондратьев В.В., 2008